Strawberry Shake in Blazor
I´m currently working on a Blazor project fetching data with StraberryShake from a HotChocolate Fusion Gateway and I'm loving it.
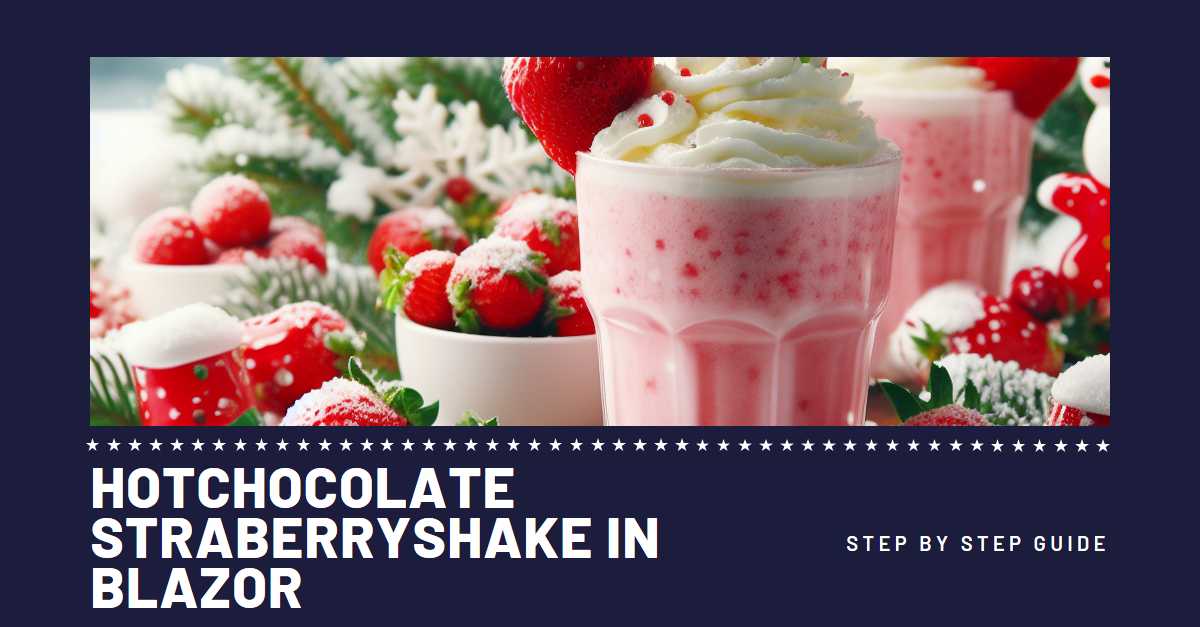
I'm currently working on a Blazor project fetching data with StraberryShake from a HotChocolate Fusion Gateway and I'm loving it.
But I had few minor issues (mostly the order of execution) getting this Get started with Strawberry Shake and Blazor article to work so I noted down what I had to do and in what order so others could have an easier time than me.
Create a tool manifest
Create a dotnet tool-manifest.
dotnet new tool-manifest
Install StrawberryShake.Tools
Install the tool
dotnet tool install StrawberryShake.Tools
Blazor project setup
Add the StrawberryShake.Blazor
nuget package
dotnet add Demo package StrawberryShake.Blazor
Btw my Blazor project is .net 7.0
and Server
(not SSR
(Static Server Rendered)). I'm using a template that needs to be updated to .net 8.0
before I can try this out with SSR
.
Have your GraphQL endpoint running
In my case it was
http://localhost:20999/graphql/
I would open up Terminal and run dotnet run
in the project root.
Create .graphqlc.json file
Do that by running the following
dotnet graphql init http://localhost:20999/graphql/ -n GatewayClient
and you should see in the output something like this
Download schema started.
Download schema completed in 916 ms
Client configuration started.
Client configuration completed in 122 ms
This will also create a schema.graphql
file. If you have issues creating the client you should make sure that your types are in there or something might have happened (it did to me but I was also using fusion)
Add namespace to .graphqlc.json
Just something like this
"namespace": "YourNamespace.Gateway"
Add some grapql query file
Add a query file like this GetCustomer.graphql
and populate it
query GetCustomers {
customer {
nodes {
Id
name
}
}
}
Nb. it needs to be a named query. That is GetCustomers
This is all of course assuming that your endpoint has customer with name and id.
Build the Blazor project
In the Blazor root run
dotnet build
and you should see
Generate C# Clients started.
Generate GatewayClient started.
Generate GatewayClient completed in 1192 ms
Generate C# Clients completed in 4019 ms
This will create GatewayClient.Client.cs
and GatewayClient.Components.cs
for you in the obj\Debug\net7.0\berry
folder.
Add the namespace to the _Import.razor file
Now you can add @using YourNamespace.Components
into the _Import.razor
for the components to show up globally in all pages desired.
Add the graphQL client
Add this code to the Program.cs
builder.Services
.AddGatewayClient()
.ConfigureHttpClient(client => client.BaseAddress = new Uri("http://localhost:20999/graphql"));
Add code to Razor page
Now you can use <UseGetCustomers>
in your Razor pages. These types are in the GatewayClient.Components.cs
file and are made up of Use+TheNameOfTheNamedQuery
<UseGetCustomers Context="result" Strategy="ExecutionStrategy.CacheFirst">
<ChildContent>
<div style="border-style: dotted">
<h3>This data is coming from the GetCustomers.graphql query</h3>
@if (result.Customer?.Nodes is not null)
{
@foreach (var customer in result.Customer.Nodes)
{
<p>@customer.Name</p>
}
}
</div>
</ChildContent>
<ErrorContent>
Something went wrong ...<br />
@result.First().Message
</ErrorContent>
<LoadingContent>
Loading ...
</LoadingContent>
</UseGetCustomers>
You should now have intellisense in you IDE.
Troubleshooting
I think I'm covering everything that happened to me but if not let me know.
Schema issue
Seeing something like the following means that you have not gotten the new schema to the schema.graphql
file in the project.
error SS0002: The field `Customer` does not exist on the type `Query`.
Already registered by another type
I had accidentally created another schema.graphql file and got this error.
error HC0065: The name `Query` was already registered by another type.
Deleting one solved the issue.
Final words
I'm just starting to play with this but like what I see.
I have bunch of questions
- How do I pass variables into these components?
- Should I use one query for each page or many?
- And if they are many will the page call once or many times?
- Caching?
- If I have many components can I communicate between them? E.g choose id from a drop down in one that fires off a query in the other one?
- Can I (and how) use paging for a grid?
- Can I use HTMX in combination with this?
So lots to explore.